|
|
Design & Methodology |
3.1 User Interface
|
The design of the system will be such
that it allows computer users from novice to expert, to navigate around the
system with ease. It must be secure, not allowing unauthorised users to
access the wrong accounts.
The best way to achieve this in Java is by creating a series of “Cards” which the applet
can swap between as necessary. The cards to be used are listed below along
with rough sketches of each card.
|
- Login (Figure 3.1)
The Login Panel hides the users password and only allows users through to main panel
after login has been validated.
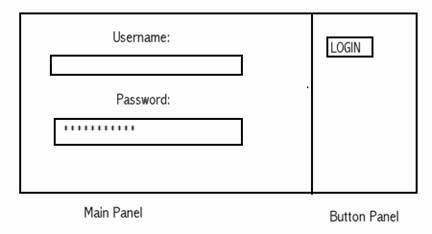
Figure 3.1 - Login Screen Design
-
Info (Figure 3.2)
The Info Panel can be called from anywhere in the program, to display
information such as error messages.
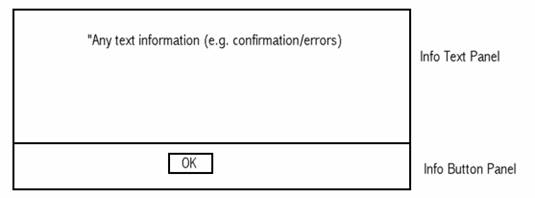
Figure 3.2 – Info Screen Design
-
Main (Figure 3.3)
The Main Panel shows how many messages are waiting for a user and allows
access to all other parts of the system.
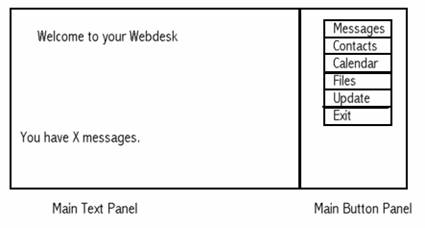
Figure 3.3 – Main Screen Design
-
Messages (Figure 3.4)
Display all messages, Update button refreshes the message list, Send
button allows the user to send a message and Delete button deletes all
messages to current user.
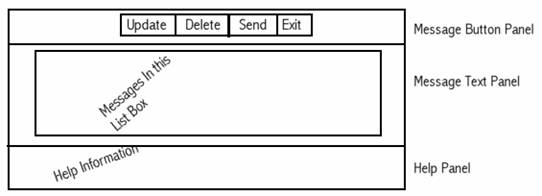
Figure 3.4 – Message Screen Design
-
Send Message (Figure 3.5)
User must address message to a particular user or the word “GROUP”
(sending to all members of users group). After sending confirmation should
be displayed.
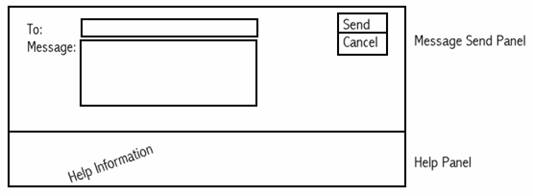
Figure 3.5 – Message Send Screen Design
- Contacts (Figure 3.6)
Screen lists Personal and Group contacts and allows user to copy between
the two.
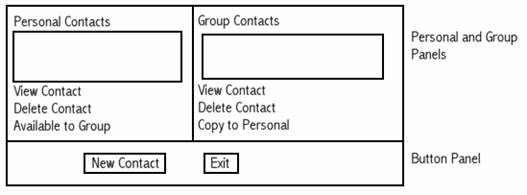
Figure 3.6 – Contacts Screen Design
- Contact Information (Figure 3.7)
Screen for entering new contact or viewing existing ones.
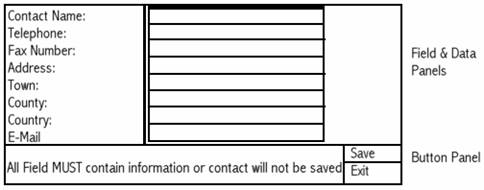
Figure 3.7 – Contact Data Screen Design
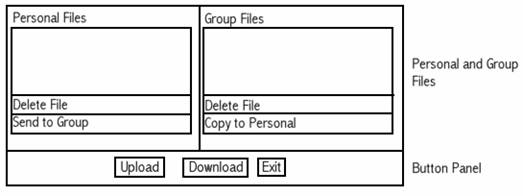
Figure 3.8 – File Screen Design
- Calendar (Figure 3.9)
Screen for storing information about meetings.
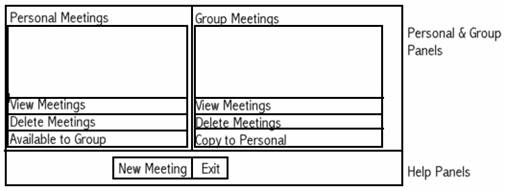
Figure 3.9 – Calendar Screen Design
- Meeting Data (Figure 3.10)
Screen for entering or viewing data about a particular meeting.
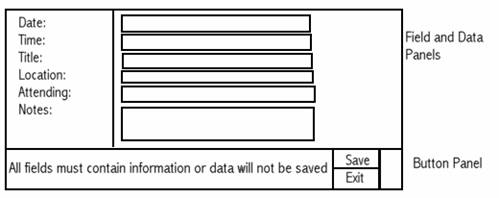
Figure 3.10 – Meeting Screen Design
|
3.2 Data
|
All data for this system will be held within a Microsoft Access database containing
various tables. Access is the chosen database because it uses the most
commonly used programming interface for relational databases – Microsoft’s
ODBC (Open DataBase Connectivity). JDBC (the standard for connecting
databases to Java) can be used with the JDBC-ODBC Bridge to connect to an
Access database, which will handle SQL (Structured Query Language)
statements well.
|
Tables to be included:
|
Table Name: |
USERS |
Data: |
Contains login and group information for
each user |
Fields: |
UName |
Text[15], No Duplicates |
|
UID |
Text[06], No Duplicates |
|
UGroup |
Text[15] |
|
UPassword |
Text[15] |
|
|
|
UName |
UID |
UGroup |
UPassword |
JBloggs |
3453 |
car_owners |
password |
|
|
Table Name: |
MESSAGES |
|
Data: |
Contains messages sent between users |
Fields: |
MSGTo |
Text[15], Indexed |
|
MSGFrom |
Text[15] |
|
MSGContent |
Text[15] |
|
|
|
MSGTo |
MSGFrom |
MSGContent |
3453 |
JSmith |
Test Message |
|
|
Table Name: |
CONTACTS |
|
Data: |
Contains user and group addresses |
Fields: |
Owner |
Text[15], Indexed |
|
Name |
Text[30] |
|
Telephone |
Text[20] |
|
Fax |
Text[20] |
|
Address |
Text[30] |
|
Town |
Text[20] |
|
County |
Text[20] |
|
Country |
Text[20] |
|
Email |
Text[30] |
|
|
|
Owner |
Name |
Telephone |
Fax |
Address |
Town |
County |
Country |
Email |
|
|
|
|
|
|
|
|
|
|
|
Table Name: |
FILES |
|
Data: |
Contains links to file in the file areas on
the server. |
Fields: |
Owner |
Text[15], Indexed |
|
FileTitle |
Text[30], Indexed |
|
FileLocation |
Text[50] |
|
|
|
Owner |
FileTitle |
FileLocation |
3453 |
Financial Report |
c:\users\files\report.doc |
|
|
Table Name: |
CALENDAR |
|
Data: |
Contains user and group appointment data. |
Fields: |
Owner |
Text[15], Indexed |
|
Date |
Date/Time, Indexed |
|
Time |
Date/Time, Indexed |
|
Title |
Text[20] |
|
Location |
Text[20] |
|
Attending |
Text[20] |
|
Notes |
Text[50] |
Owner |
Date |
Time |
Title |
Location |
Attending |
Notes |
3453 |
12/11/2000 |
13:00 |
AGM |
Grand Hotel |
All Employees |
Casual |
|
|
Each of the data structures above will be
represented as tables in Microsoft Access and these tables can therefore
mirrored as classes in Java (with JDBC). Making the transfer from
Microsoft Access to Java very easy.
|
|
3.3 Structure
|
The structure of the movement between cards is shown in
Figure 3.11. |
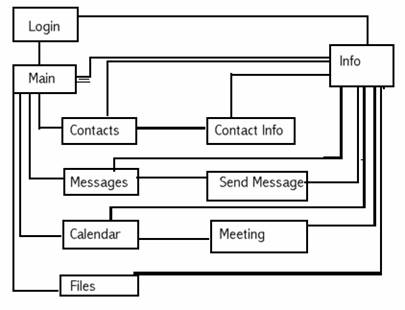
Figure 3.11 - Card Structure Design
|
Each of these cards will be held as part of a card panel,
which along with a layout controller class will control the movement between
screens. The information panel shown must always be able to return to the
screen from which it was called.
The Server must be multithreaded, to allow multiple users
to read and write to the database at any one time (the intial version should
have a maximum of 10 threads/users), and should display what each thread is
doing on the screen, to allow monitoring of user activity.
The client should not contain a continuous connection to
the database, this allows for other users to access and to avoid data
corruption if the Internet connection is lost. So the client must log in to
the database, perform what ever is necessary and logout whenever necessary.
|
3.4 Classes and Pseudocode
|
Pseudocode - MyWebDeskClient (Extension of Applet)
Init() |
Set applet size, create card panels and CurrentUser. |
Start() |
Create RMI reference to the database. |
PadString(String, int) |
Utility function for padding strings to a length with spaces. |
ShowMessage(String) |
Use the Information Screen to display a message. |
|
Do NOT make the information panel the current screen. |
updateScreens(String) |
Update
specified screens in system. |
ContactData(String) |
Retrieve a contact and place details in contact details screen. |
MeetingDate(Meeting) |
Retrieve a meeting and place details in meeting details screen. |
|
|
Class: CardPanel (Extension of Panel)
CardPanel() Constructor |
Create all Panels, set Login Screen to be displayed |
setMsg(String) |
Set message to be displayed on information screen. |
update(String) |
Update screens specified with data for current user |
aContactUpdate(Contact) |
Update Contact details screen with relevant data. |
aMeetingUpdate(Meeting) |
Update Meeting details screen with relevant data. |
|
|
Class: MyWebDeskScreensLayout (Extension of CardLayout)
|
MyWebDeskScreensLayout(String)
Constructor |
|
Creates Layout Manager and sets its current screen. |
setCurrent(String) |
Sets Current Screen. |
show(Container, String) |
Show Screen and set to current screen. |
show(Container, String, Boolean) |
|
Show Screen and set to current if necessary. |
showCurrent(Container) |
Show Current Screen. |
|
|
Class: LoginPanel (Extension of Panel, Implementation of ActionListener)
LoginPanel() Constructor |
Creates Login Screen (as shown in Figure 3.1). |
|
Ensure character for password is '*'. |
getLogin() |
Returns the users ID who is attempting Login. |
getPassword() |
Returns the users password who is attempting Login. |
Login(String, String) |
Set Login to False. |
|
Check Login Details in Database. |
|
If passwords match, set Current User and login returns
true. |
ActionPerformed (ActionEvent) |
|
"Login" clicked. Attempt to login. |
|
If login is valid then update all screen with the users
data and set the screen to main. |
|
If login is invalid inform user and return to login
screen. |
|
|
Class: InfoPanel (Extension of Panel, Implementation of ActionListener)
InfoPanel() Constructor |
Creates Information Screen (as shown in Figure 3.2). |
setMsg(String) |
Sets the message on the information screen. |
ActionPerformed (ActionEvent) |
|
"OK" clicked. Return to previous screen. |
|
|
Class: InfoPanel (Extension of Panel, Implementation of ActionListener)
MainPanel() Constructor |
Creates Main Screen (as shown in Figure 3.3). |
Update() |
Searches database for messages and for current user. |
|
Displays results on Main Screen. |
ActionPerformed (ActionEvent) |
|
Display appropriate screen based upon button press. |
|
|
Class: MessagePanel (Extension of Panel, Implementation of
ActionListener)
MessagePanel() Constructor |
|
Creates Message Screen (as shown in Figure 3.4). |
Update() |
Search Database for messages for current user. |
|
Display in list box on Message Screen. |
Delete() |
Delete all messages in database for current user. |
ActionPerformed (ActionEvent) |
|
"Update" clicked, call Update().
"Delete" clicked, call Delete().
"Send" clicked, show SendMessage Screen.
"Cancel" clicked, show MainScreen. |
|
|
Class: SendMsgPanel (Extension of Panel, Implementation of
ActionListener)
sendMsgPanel() Constructor |
|
Creates Send Message Screen (as shown in Figure 3.5). |
getMsgTo() |
Return the recipient entered by user. |
getMsgContent() |
Return the message being sent. |
getID(String) |
Return the User ID of the recipient from the database. |
SendPersonalMsg(String, Message) |
|
Add the message to the table of messages. |
SendGroupMsg(String, Message) |
|
Check for members of the group and perform
SendPersonalMsg for each. |
ActionPerformed (ActionEvent) |
|
"Cancel" clicked, return to messages screen.
"Send" clicked, check if group or personal message
If a group message call SendGroupMsg() otherwise check user exists and
call SendPersonalMsg().
Show Information Screen, how many messages were sent. |
|
|
Class: ContactsPanel (Extension of Panel, Implementation of
ActionListener)
ContactsPanel() Constructor |
|
Create Contacts Screen (as shown in Figure 3.6). |
Update() |
Fill Personal and Group contact lists with contacts. |
View(String, String) |
Fill aContact screen with data to be reviewed. |
Delete(String, String) |
If executed on a personal contact, delete personal
contact. |
|
If executed on a group contact, delete group contact.
Call Update(). |
Swap (String, String) |
Make a copy of contact and give ownership to user or
group. |
ActionPerformed (ActionEvent) |
|
"New Contact" clicked, show blank aContact Screen.
"View Contact" clicked, show filled aContact Screen.
"Delete Contact" clicked, call Delete().
"Available to Group" clicked, call Swap().
"Copy to Personal" clicked, call Swap(). |
|
|
Class: aContactPanel (Extension of Panel, Implementation of
ActionListener)
aContactPanel() Constructor |
|
Create aContact Screen (as shown in Figure 3.7). |
Empty() |
Empty Screen of old data. |
FillData(Contact) |
Fill Screen with contact data returned from query. |
Save() |
Save current details in Database. |
ActionPerformed (ActionEvent) |
|
"Save" clicked, call Save()
"Exit" clicked, show Contacts Screen |
|
|
Class: FilesPanel (Extension of Panel, Implementation of ActionListener)
FilesPanel() Constructor |
Creates Files Screen (as shown in Figure 3.8) |
Update() |
Update Personal and Group file lists. |
Upload(String, String) |
FTP Receive file and create a record in the database. |
Download(String, String) |
FTP Download file. |
Delete() |
Delete file from server and remove a record of it from
database. |
MakeGroup(String, String) |
Create a copy of file and place in group file area. |
|
Create a copy of record and change owner to name of
group. |
MakePersonal(String,String) |
Create a copy of file and place in personal file area. |
|
Create a copy of record and change owner to User ID. |
ActionPerformed (ActionEvent) |
|
"Update" clicked, call Update.
"Upload" clicked, call Upload.
"Download" clicked, call Download.
"Delete" clicked, call Delete.
"Allow Group Access" clicked, call MakeGroup.
"Make a Personal Copy" clicked, call MakePersonal. |
|
|
Class: MeetingPanel (Extension of Panel, Implementation of
ActionListener)
MeetingsPanel() Constructor |
|
Create Meetings Screen (as shown in Figure 3.9). |
Update() |
Fill personal and group lists with data as required. |
View(String, String) |
Fills a Meeting Screen with data to be viewed. |
Delete(String, String) |
Deletes a meeting. |
Swap(String, String, String) |
Makes a copy of a meeting and gives ownership to group
or personal necessary. |
ActionPerformed (ActionEvent) |
|
"View Meeting" clicked, call filled aMeeting Screen.
"Delete Meeting" clicked, call Delete().
"Available to Group" clicked, call Swap().
"Make a Personal Copy" clicked, call Swap().
"New Meeting" clicked, call aMeeting Screen.
"Exit" clicked, call Main Screen. |
|
|
Class: MeetingPanel (Extension of Panel, Implementation of
ActionListener)
aMeetingPanel() Constructor |
|
Create aMeeting Screen (as shown in Figure 3.10). |
Empty() |
Empty screen of old data. |
FillData(Meeting) |
Fill with Meeting being returned from query. |
Save() |
Saves a Meeting into the Meeting table. |
ActionPerformed (ActionEvent) |
|
"Exit" clicked, Meetings screen.
"Save" clicked, Save data and return to Meetings screen. |
|
|
Pseudocode - MyWebDeskThread (Extension of Thread)
Class: MyWebDeskThread() (Extension of Thread)
run() |
Hold the thread open so that remote objects can call its
methods. |
openMyWebDesk() |
Open the database for connection using JDBC-ODBC Bridge. |
closeMyWebDesk() |
Delete all old results, statements and connections. |
searchMyWebDesk(String) |
Perform an SQL query on MyWebDesk Database. |
UpdateMyWebDesk(String) |
Perform an SQL update on MyWebDesk Database. |
getNextUser() |
If there is another user in the results set, create as a
new object and return it. Else return null. |
getNextMessage() |
If there is another message in the results set, create
as a new object and return it. Else return null. |
getNextContact() |
If there is another contact in the results set, create
as a new object and return it. Else return null. |
getNextMeeting() |
If there is another meeting in the results set, create
as a new object and return it. Else return null. |
|
|
Pseudocode - MyWebDeskServer (Application)
Main() |
Set Security Manager. |
|
Bind Server to RMI Registry. |
OpenMyWebDesk() |
Find an empty thread and return to user. |
|
Create a new MyWebDeskThread for user and start it. |
|
Call openMyWebDesk() for new thread. |
CloseMyWebDesk() |
Call closeMyWebDesk() for thread. |
|
Stop the thread. |
|
Create pointer from thread to null. |
SearchMyWebDesk() |
Call searchMyWebDesk() for thread. |
UpdateMyWebDesk() |
Call updateMyWebDesk() for thread. |
getNextUser() |
Return next user for thread. |
getNextMessage() |
Return next message for thread. |
getNextContact() |
Return next contact for thread. |
getNextFile() |
Return next file for thread. |
getNextMeeting() |
Return next meeting for thread. |
|
|
|